-----
1) Hello World Program
2) Sum Numbers Program
3) Sum Variables Program
4) Multiply Variables Program
5) Divide Variables Program
7) Display Array Values Program
1) Hello World Program
-----
Flow Chart:
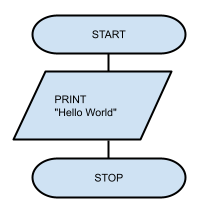
-----
Pseudo Code:
1. BEGIN
2. PRINT "Hello World"
3. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. PRINT "Hello World"
3. END
-----
C Language sample code:
-----
C Language sample output:
2) Sum Numbers Program
-----
Flow Chart:
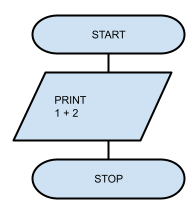
----
Pseudo Code:
1. BEGIN
2. PRINT 1 + 2
3. END
-----
C Language sample code:
-----
C language sample output:

Flow Chart:
----
Pseudo Code:
1. BEGIN
2. PRINT 1 + 2
3. END
-----
C Language sample code:
-----
C language sample output:
3) Sum Variables Program
-----
Flow Chart:
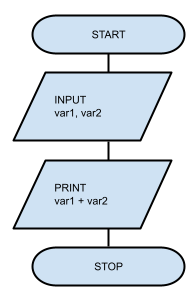
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. PRINT var1+var2
4. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. PRINT var1+var2
4. END
-----
C Language sample code:
-----
C Language sample output:
4) Multiply Variables Program
-----
Flow Chart:
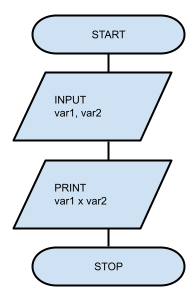
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. PRINT var1 x var2
4. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. PRINT var1 x var2
4. END
-----
C Language sample code:
-----
C Language sample output:
5) Divide Variables Program
-----
Flow Chart:
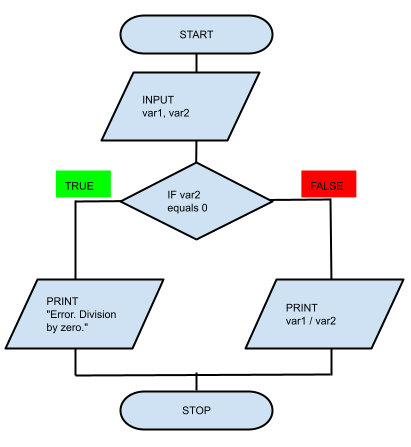
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. IF var2=0
4. THEN PRINT "Error. Division by zero."
5. ELSE PRINT var1/var2
6. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. INPUT var1, var2
3. IF var2=0
4. THEN PRINT "Error. Division by zero."
5. ELSE PRINT var1/var2
6. END
-----
C Language sample code:
-----
C Language sample output:
6) Count Numbers Program
-----
Flow Chart:
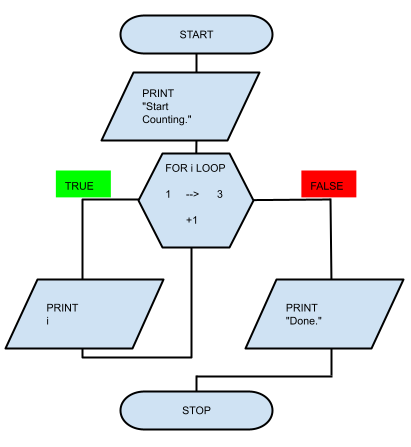
-----
Pseudo Code:
1. BEGIN
2. PRINT "Start counting."
3. FOR i LOOP := 1 to 3
4. PRINT i
5. END LOOP
6. PRINT "Done."
7. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. PRINT "Start counting."
3. FOR i LOOP := 1 to 3
4. PRINT i
5. END LOOP
6. PRINT "Done."
7. END
-----
C Language sample code:
-----
C Language sample output:
7) Display Array Values Program
-----
Flow Chart:
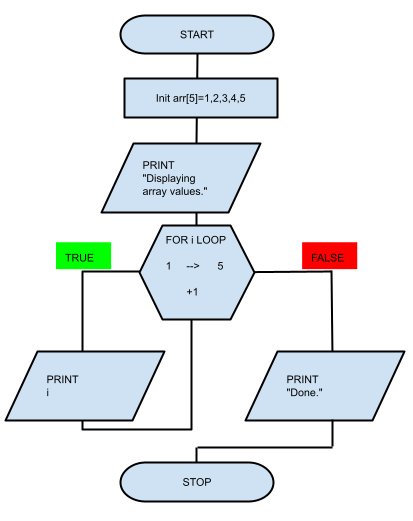
-----
Pseudo Code:
1. BEGIN
2. INITIALIZE ARRAY arr[5] TO CONTAIN VALUES 1,2,3,4,5
3. PRINT "Displaying array values."
4. FOR i := 1 to 5
5. PRINT arr[i]
6. END FOR LOOP
7. PRINT "Done."
8. END
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. INITIALIZE ARRAY arr[5] TO CONTAIN VALUES 1,2,3,4,5
3. PRINT "Displaying array values."
4. FOR i := 1 to 5
5. PRINT arr[i]
6. END FOR LOOP
7. PRINT "Done."
8. END
-----
C Language sample code:
-----
C Language sample output:
8) Edit Array Values Program
-----
Note:
This tutorial demonstrates the steps to edit an array value. The program displays the array values before and after the edit is done. There are code redundancies here. Ignore this for now.
-----
Flow Chart:
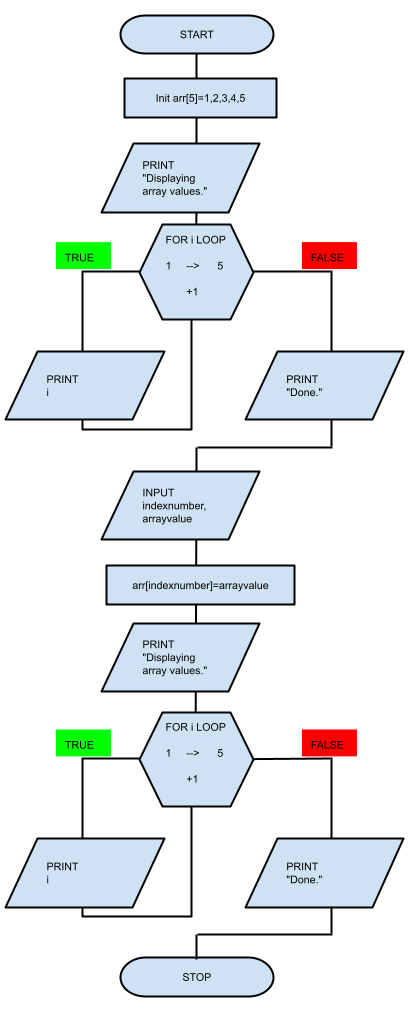
-----
Pseudo Code:
1. BEGIN
2. INITIALIZE ARRAY arr[i] WITH VALUES 1,2,3,4,5
3. FOR i := 1 to 5
4. PRINT arr[i]
5. END FOR LOOP
6. INPUT indexnumber
7. INPUT arrayvalue
8. arr[indexnumber] = arrayvalue
9. FOR i := 1 to 5
10. PRINT arr[i]
11. END FOR LOOP
C Language sample code:
-----
C Language sample output:

Note:
This tutorial demonstrates the steps to edit an array value. The program displays the array values before and after the edit is done. There are code redundancies here. Ignore this for now.
-----
Flow Chart:
-----
Pseudo Code:
1. BEGIN
2. INITIALIZE ARRAY arr[i] WITH VALUES 1,2,3,4,5
3. FOR i := 1 to 5
4. PRINT arr[i]
5. END FOR LOOP
6. INPUT indexnumber
7. INPUT arrayvalue
8. arr[indexnumber] = arrayvalue
9. FOR i := 1 to 5
10. PRINT arr[i]
11. END FOR LOOP
12. END
-----C Language sample code:
-----
C Language sample output:
9) Modular Program
-----
Flow Chart:
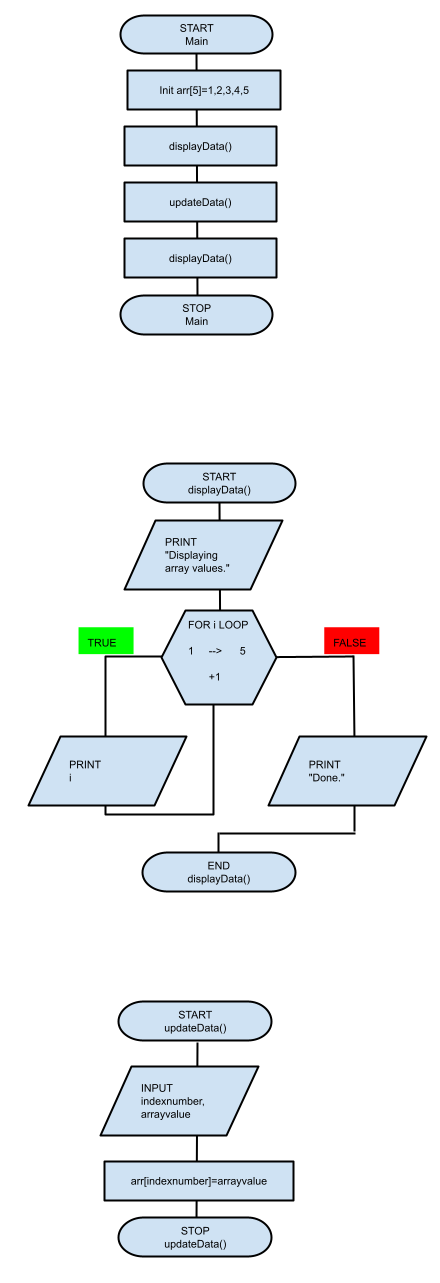
-----
Pseudo Code:
1. BEGIN main
2. CALL displaydata
3. CALL updatedata
4. CALL displaydata
5. END main
1. BEGIN displaydata
2. FOR all item in array PRINT item values
3. END displaydata
-----
C Language sample code:
-----
C Language sample output:

Flow Chart:
-----
Pseudo Code:
1. BEGIN main
2. CALL displaydata
3. CALL updatedata
4. CALL displaydata
5. END main
1. BEGIN displaydata
2. FOR all item in array PRINT item values
3. END displaydata
1. BEGIN updatedata
2. INPUT indexnumber, arrayvalue
3. arr[indexnumber]=arrayvalue
2. INPUT indexnumber, arrayvalue
3. arr[indexnumber]=arrayvalue
4. END updatedata
-----
C Language sample code:
-----
C Language sample output:
No comments:
Post a Comment